This article explains how I used Xdebug to debug a cURL request made from one WordPress installation to another on the same local server.
Although I personally use PhpStorm as an editor, the things covered in this article are not limited to any specific editor, so as long as you have Xdebug working for standard requests, you should be able to follow everything. If you don’t have this already set up, here’s a tutorial that should get you something that works, and then you can come back here: https://medium.com/@michalisantoniou6/set-up-xdebug-with-homestead-phpstorm-and-php7-85b5ac8f0c79
Xdebug in action
The problem I had was that I was trying to debug both websites at the same time, with one of the requests being made from one remote website to the other. Below is what I started with as a configuration.
xdebug.remote_enable = 1
xdebug.remote_connect_back = 1
xdebug.remote_port = 9000
The xdebug.remote_enable
has to be open. In my case, I used Vagrant for my development machine, so it was actually a connection from machine to Vagrant.
xdebug.remote_connect_back
will make Xdebug try to connect back to the request IP. To do so, it uses the $_SERVER['HTTP_X_FORWARDED_FOR']
and $_SERVER['REMOTE_ADDR']
The xdebug.remote_port
option doesn’t affect this flow – just make sure it’s the same as the port set in your editor.
This wasn’t working in my case because the remote address was not the one from my computer (the host), but rather the one from Vagrant. This differs from when you try to debug a single website via browser calls, as in this instance, you set the remote address as the computer on which you have the editor opened. On the other hand, if you call it via cURL, it will try to connect back to the Vagrant machine, which has no listener for Xdebug.
In order to fix this, you must, therefore, make sure that Xdebug is indeed connecting back to your machine and not to the one that made the cURL request, since you have the editor open and listening for that Xdebug connection.
So how do we do that? This is the code you need in your cURL request:
curl_setopt($ch, CURLOPT_HTTPHEADER, array(
'X-Xdebug-Remote-Address: '.$_SERVER['REMOTE_ADDR'],
'Cookie: XDEBUG_SESSION=PHPSTORM'
));
You first need to forward your browser request IP to the server so that it will return that Xdebug call to your computer. Second, you need your IDE to connect the session to your project, as you will need to send the session with the key that the editor created when you started the debug session. In other words, replace PHPSTORM in the example with the key you get when you press the debug button: http://example.local/?XDEBUG_SESSION_START=15342
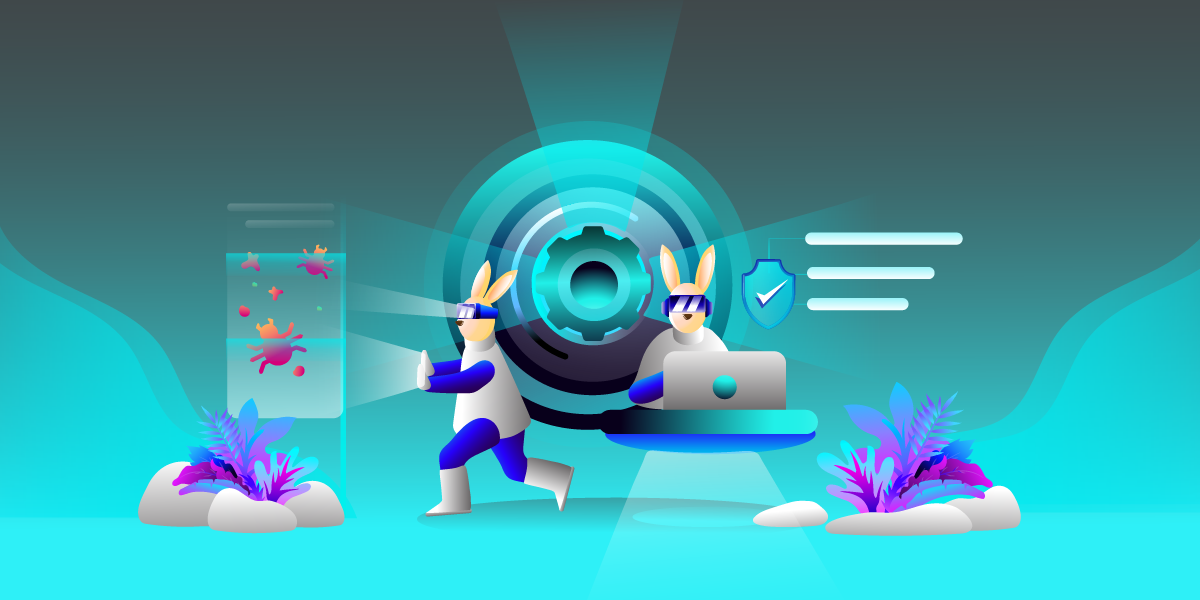
That’s it, you should then be good to go – it will now connect the debug session to your project. This works even if you don’t have your project on Vagrant and is all local, as the core idea is the same.